Joined November 2013
·
Posted to
Javascript inheritance
over 1 year
ago
I think with this example you can create hierarchies easier.
jsfiddle
function ex (destination, source) {
var p;
for (p in source) {
if (source.hasOwnProperty(p)) {
destination[p] = source[p];
}
}
return destination;
}
function extend (data, staticData) {
var parent = this,
child = function () {
if (data.initialize) {
data.initialize.apply(this, arguments);
} else {
parent.apply(this, arguments);
}
};
ex(child, parent);
if (staticData) {
ex(child, staticData);
}
if (!data) {
data = {};
}
child.prototype = ex(Object.create(parent.prototype), data);
child.prototype.constructor = child;
child.prototype.__super = parent.prototype;
return child;
}
function Foo (a, b) {
this.a = a;
this.b = b;
}
Foo.prototype.c = function () {
return this.a + this.b
};
var foo = new Foo(2, 5);
console.log(foo.c());
Foo.extend = extend;
Bar = Foo.extend({
initialize: function (a, b, d) {
this.__super.constructor(a, b);
this.d = d;
},
d: 8,
e: function () {
return this.a + this.b + this.d;
}
});
var bar = new Bar(3, 1, 9);
console.log(bar.c(), bar.e());
var Baz = Bar.extend({
initialize: function (a, b, d, f) {
this.__super.constructor(a, b, d);
this.f = f;
},
f: 2,
g: function () {
return this.a + this.b + this.d + this.f;
}
});
var baz = new Baz(3, 1, 4, 6);
console.log(baz.c(), baz.e(), baz.g());
Achievements
116 Karma
7,145 Total ProTip Views
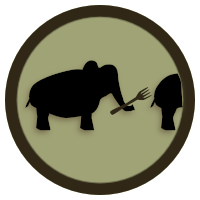
Forked
Have a project valued enough to be forked by someone else
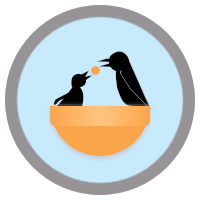
Charity
Fork and commit to someone's open source project in need
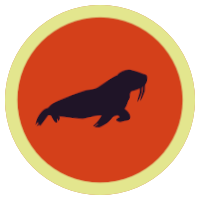
Walrus
The walrus is no stranger to variety. Use at least 4 different languages throughout all your repos
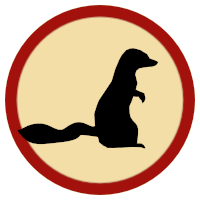
Mongoose
Have at least one original repo where Ruby is the dominant language
Seems too complex to me :)