Swift
let Christmas="beer "
let NewYear="more beer"
if Christmas+NewYear=="beer more beer" {
println("festive hangover")
}
I've not worked through these additions yet https://developer.apple.com/library/ios/documentation/Cocoa/Conceptual/AttributedStrings/Articles/HTMLFilesandAttributedStrings.html#//apple_ref/doc/uid/TP40014062-SW1 and I think they are iOS 8 only
Taking a brief look at StackOverflow, it looks like the thing you are asking isn't possible - e.g. http://stackoverflow.com/questions/1262574/add-uipickerview-a-button-in-action-sheet-how - and that you need to find some other way around the problem.
Thanks for the comment. To explain, this isn't triggering a storyboard segue, this is instantiating a view controller that has been created visually in interface builder (or whatever you want to call that area of Xcode now).
This is like C64 Big Mouth all over again, thanks for sharing
You might need to Redirect and Rewrite (and in the right order), the best thing to do is to look at the modrewrite documentation: http://httpd.apache.org/docs/2.2/mod/modrewrite.html and http://httpd.apache.org/docs/2.2/rewrite/remapping.html
Try this instead:
## 301 Redirect Entire Directory
RedirectMatch 301 teamfront.php?(.*) /$1
@prakash j: You seem to be talking about a redirect rather than a rewrite, I've not implemented any myself but I think you'd need to do something like this in your .htaccess file
## 301 Redirect Entire Directory
RedirectMatch 301 http://tresbizz.com/teamfront.php?(.*) http://tresbizz.com/$1
@prakash j you shouldn't need all the http://www.sub.myurl.com because you'll just be redirecting from either the base folder or a subfolder.
The first part - ^([a-zA-Z0-9]+|)/?$ - is the regex that captures the input text. We then reference the text captured by the regex with $1 and send it to index.php using the command index.php?name=$1.
In your example you've missed out various bits, it should look more like this:
RewriteEngine on
RewriteRule ^([a-zA-Z0-9]+|)/?$ viewtopic.php?product_names=$1
and this should be saved in a .htaccess file in the base folder of your site: http://www.sub.myurl.com/
You then will be able to capture the product name using:
<?php echo $_GET['product_names']; ?>
in the viewtopic.php file when the URL is something like this: http://www.sub.myurl.com/catfood
I wish someone would tell the IDPF, who create the EPUB standard, this. Metadata would be greatly simplified with JSON.
@harryfinn I'm glad that it helped you out, and thanks for taking the time to comment.
Not working with hyperlinks, because you don't close the <a></a> tags in your first two preg_replace calls
function twitterify($ret) {
$ret = preg_replace("#(^|[\n ])([\w]+?://[\w]+[^ \"\n\r\t< ]*)#", "\\1<a href=\"\\2\" target=\"_blank\">\\2</a>", $ret);
$ret = preg_replace("#(^|[\n ])((www|ftp)\.[^ \"\t\n\r< ]*)#", "\\1<a href=\"http://\\2\" target=\"_blank\">\\2</a>", $ret);
$ret = preg_replace("/@(\w+)/", "<a href=\"http://www.twitter.com/\\1\" target=\"_blank\">@\\1</a>", $ret);
$ret = preg_replace("/#(\w+)/", "<a href=\"http://twitter.com/search?q=\\1\" target=\"_blank\">#\\1</a>", $ret);
return $ret;
}
@shogun I think that's a really good question. The example is part of a tutorial and really intended for the insertion of functions to process the objects depending on type, not just to output the name, as it is doing now.
I like the switch statement for its readability, and the continued readability when functions are inserted, but I'm not a JS expert, and consider myself a forever-learning user, so I am grateful for guidance in these areas.
I've discovered that you can create Base64 images using OpenSSL in the OS X Terminal app - all is explained here http://sketchytech.blogspot.co.uk/2012/12/base64-encoding-of-image-files.html
Achievements
3,568 Karma
476,068 Total ProTip Views
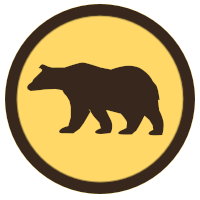
Bear
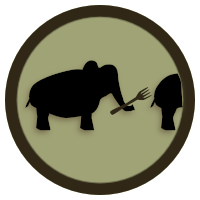
Forked
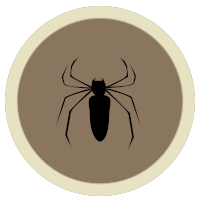
The first line doesn't do anything in this example and stringWithContentsOfFile is deprecated. To print the string, you'd do this:
but if you wanted to transform the JSON into data you'd do something like this:
However, downcasting to a dictionary or an array doesn't transform all nested content into Swift instances and so it is a process that will need to be repeated. Hence the Swift/JSON discussions and GitHub postings that have been going on.