Joined September 2011
·
Achievements
138 Karma
5,428 Total ProTip Views
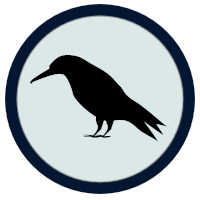
Raven
Have at least one original repo where some form of shell script is the dominant language
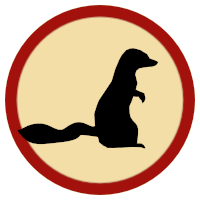
Mongoose
Have at least one original repo where Ruby is the dominant language
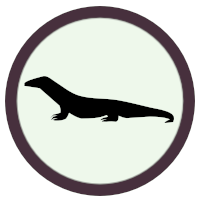
Komodo Dragon
Have at least one original repo where Java is the dominant language
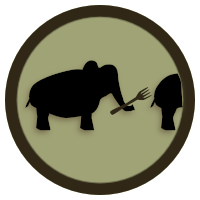
Forked
Have a project valued enough to be forked by someone else
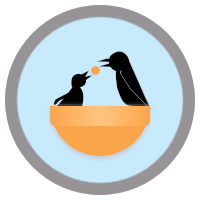
Charity
Fork and commit to someone's open source project in need
Don't you ever thought about using bind to "hold over" a function call and make your code look nice w/o any libs. Except using an internal Function.bind() (not in all browsers), you may write a 5-liner function like this one, the one which will "defer" calls to the function it wraps:
It will allow you to write something like this:
It is just a draft to give a closer look to idea, different implementations will require different ways to call/implement it (i.e. partial functions, where you pass only some part of parameters in call and joining them with the ones left while doing a second call, like in Scala; btw, Function.bind() allows this, again), but I really doubt that proper variants of these implementations will take the same amount of KBs from user as most of those libs do — most of them are few-liners. Be monadic ;).