Joined November 2013
·
Posted to
More readable git word diff on macOS or Amazon Linux/CentOS/RedHat
over 1 year
ago
I prefer just setting it in the global config without modifying $PATH
. It's already a mess.
And it's all CLI.
git config --global pager.log `brew --prefix`/share/git-core/contrib/diff-highlight/diff-highlight
git config --global pager.show `brew --prefix`/share/git-core/contrib/diff-highlight/diff-highlight
git config --global pager.diff `brew --prefix`/share/git-core/contrib/diff-highlight/diff-highlight
Posted to
Rails - filter using join model on has_many through
over 1 year
ago
Here's a full rails test-like gist: https://gist.github.com/tzvetkoff/7287456
Posted to
Rails - filter using join model on has_many through
over 1 year
ago
OK, everything is cool but... why don't you use Rails's STI
& default_scope
s for that?
Besides the really nice query interface, you'll also get the write association methods working for free, as ActiveRecord can populate default values from the current scope.
# The person, a real object
class Person < ActiveRecord::Base
# Every computer that person has access to
has_many :accounts
has_many :computers, :through => :accounts
# Only comps that this account can administrate
has_many :administrator_accounts, :class_name => 'Account::Administrator'
has_many :administrated_computers, :through => :administrator_accounts, :source => :computer
end
# The account - a relationship (<3) between a person and a computer
class Account < ActiveRecord::Base
belongs_to :person
belongs_to :computer
# The administrator subclass - here we're using a `default_scope` rather than just normal STI with a `type` field (which I prefer)
class Administrator < Account
default_scope lambda { where(:role => 'administrator') }
end
end
# The computer being used
class Computer < ActiveRecord::Base
# [ALL] Users
has_many :accounts
has_many :people, :through => :accounts
# Administrators through abnormal accounts
has_many :administrator_accounts, :class_name => 'Account::Administrator'
has_many :administrators, :through => :administrator_accounts, :source => :person
end
person1 = Person.create
person2 = Person.create
comp1 = Computer.create
comp2 = Computer.create
person1.computers << comp1
person1.administrated_computers << comp2
person2.computers << comp2
person2.administrated_computers << comp1
ap person1.computers.to_a
ap person1. administrated_computers.to_a
ap person2.computers.to_a
ap person2. administrated_computers.to_a
Achievements
121 Karma
0 Total ProTip Views
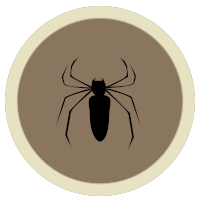
Nephila Komaci
Have at least one original repos where PHP is the dominant language
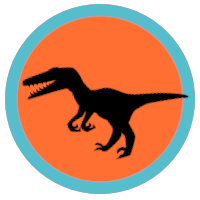
Velociraptor
Have at least one original repo where Perl is the dominant language
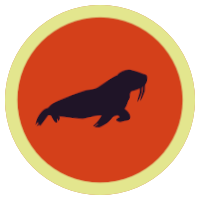
Walrus
The walrus is no stranger to variety. Use at least 4 different languages throughout all your repos
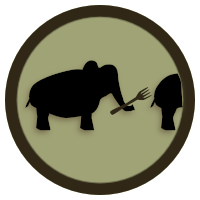
Forked
Have a project valued enough to be forked by someone else
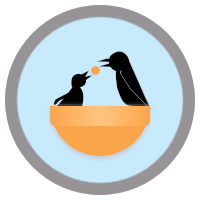
Charity
Fork and commit to someone's open source project in need
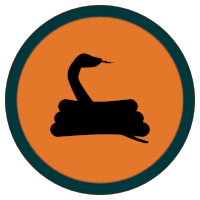
Python
Would you expect anything less? Have at least one original repo where Python is the dominant language
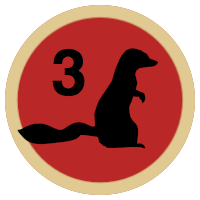
Mongoose 3
Have at least three original repos where Ruby is the dominant language
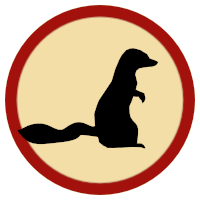
Mongoose
Have at least one original repo where Ruby is the dominant language
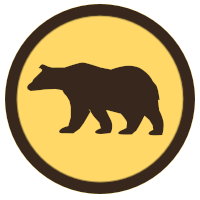
Bear
Have at least one original repo where Objective-C is the dominant language
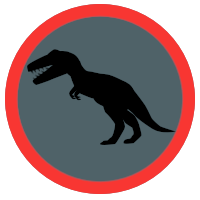
T-Rex
Have at least one original repo where C is the dominant language
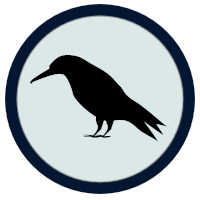
Raven
Have at least one original repo where some form of shell script is the dominant language
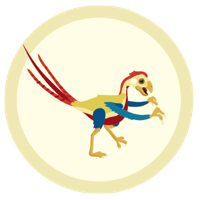
Epidexipteryx
Have at least one original repo where C++ is the dominant language
Worth noting that if you're going to use ENUMs you'll need a migration for them:
Also, a nicer core_ext/initializer for patching ActiveRecord::ConnectionAdapters::Column and adding type aliases could be put in place:
This way everything sums up to just 2 files (or more, if you need to add more ENUMs as the project goes)
And last, but not least - typecasting the value is optional: