Closures are really helpful. Now I'm not a javascript developer, so instead I present psuedo-code.
Consider you wanted a function, that you could pass in some accumulator object, whose value would be taken and applied to each iteration of the block evaluated against. So for instance:
[1,2,3,4,5].reduce(1, function(a, idx, b) { /* a is the accumulator, initially 1, b is the current element while iterating the list */ return a * b; });
This produces a single result when it returns: 120 (astute readers will realize this is an implementation of factorial).
It could be implemented like this:
function reduce(acc, fn) {
for(var i = 0; i < this.length; i++) {
acc = fn(acc, i, this[i]);
}
return acc;
}
Now let's say we wanted to apply a transformation to each object in the list, in this case, let's say squaring numbers to keep it simple, we could implement a function in terms of reduce
above
function map(fn) {
return this.reduce(new Array(this.length), function(acc, idx, elem) {
acc[idx] = fn(elem);
return acc;
}
}
This will let you calculate the square numbers from a sequence of numbers 1..n where in this example I'll define n as being equal to 5, like so:
[1,2,3,4,5].map(function(elem) { return elem * 2; })
The output of which will be this:
[2,4,6,8,10]
It doesn't take much to realize how these simple primitive operations I've defined above can be used on all sorts of data types to yield some wonderfully elegant and flexible code.
Full disclosure, I have not proven the above code correct in any language, but it should be "close enough" in many languages to be useful. Again, I'm not a Javascript programmer.
@bashir That trick is heavily dependent on your editor.
Achievements
201 Karma
2,444 Total ProTip Views
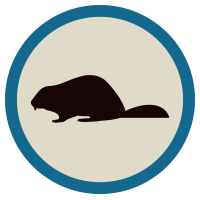
Beaver
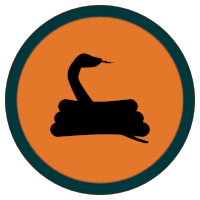
Python
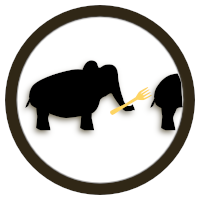
Forked 50
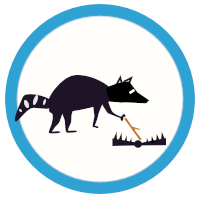
Altruist
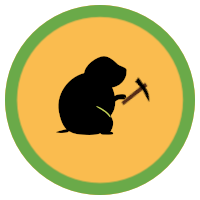
Lemmings 100
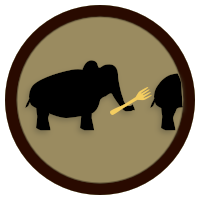
Forked 20
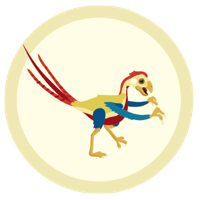
Epidexipteryx
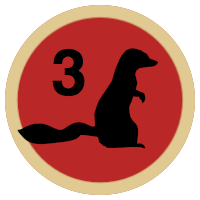
Mongoose 3
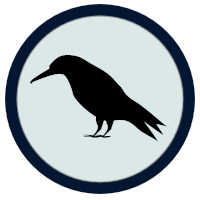
Raven
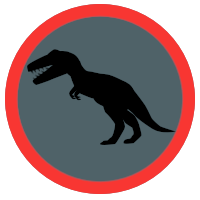
T-Rex
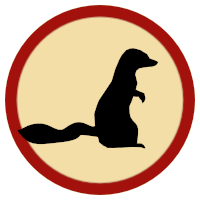
Mongoose
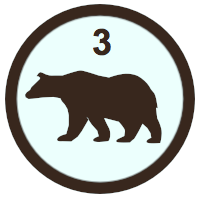
Bear 3
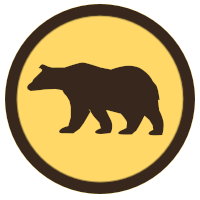
Bear
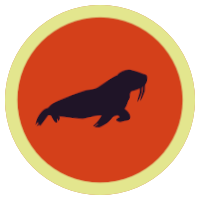
Walrus
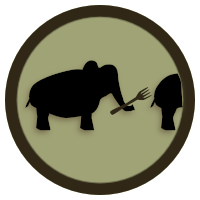
Forked
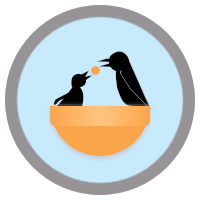
Just use a semaphore.
Just make sure to define
TIMEOUT
to some number of seconds you're willing to wait before timing out.Also none of this is needed if this "inline block" is executed synchronously on the same thread that called the method it was passed to before that method exits. I.e., the block evaluation blocks the method return until the block returns at least.