Joined July 2012
·
Posted to
"Callback Hell" in JS/Node.js is a Myth
over 1 year
ago
I'd recommend something like Q because it's compatible with ES6 generators. That means that once the next version of JS comes out you'll be able to write code like:
spawn(function* () {
var result = yield webRequestAsync('http://foo.com');
//Do something with result
console.log(JSON.parse(result).name);
});
which looks just like the sync equivalent, except for the yield keyword. It would then be exactly equivalent to:
function () {
return webRequestAsync('http://foo.com')
.then(function (result) {
//Do something with result
console.log(JSON.parse(result).name);
});
}();
Consider that yield also works perfectly inside try catch statements etc. and you're onto a winner.
Achievements
122 Karma
946 Total ProTip Views
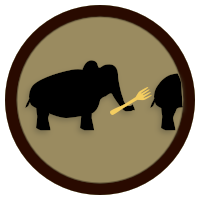
Forked 20
Have an established project that's been forked at least 20 times
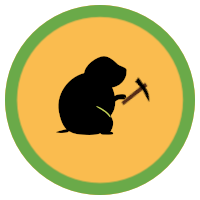
Lemmings 100
Write something great enough to have at least 100 watchers of the project
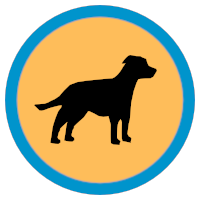
Lab
Have at least one original repo where C# is the dominant language
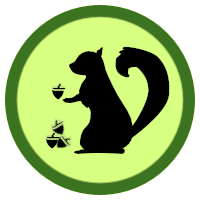
Philanthropist
Truly improve developer quality of life by sharing at least 50 individual open source projects
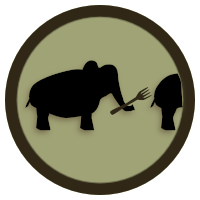
Forked
Have a project valued enough to be forked by someone else
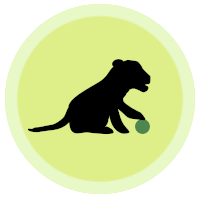
Cub
Have at least one original jQuery or Prototype open source repo
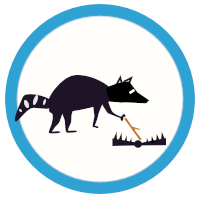
Altruist
Increase developer well-being by sharing at least 20 open source projects
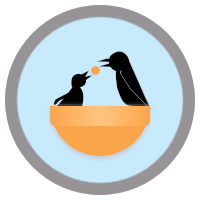
Charity
Fork and commit to someone's open source project in need
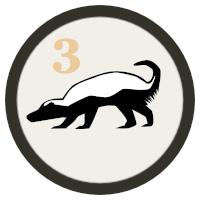
Honey Badger 3
Have at least three Node.js specific repos
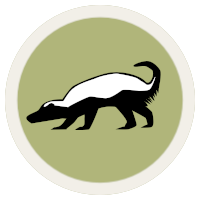
Honey Badger
Have at least one original Node.js-specific repo
Your package.json could be written as:
and would behave the same because npm is super clever