It's useful in some cases. It's just a different pattern where you have a function that can take arguments of many types. For example the jQuery constructor could be done with overloading as it takes an element, an array, a pseudo-array, a jQuery collection, a string, etc... Here's a simple example:
var double = overload({
String: function(x) {
return x + x;
},
Number: function(x) {
return x * 2;
}
});
console.log(double('hey')); //=> heyhey
console.log(double(9)); //=> 18
You can use multiple if
statements to check the type and the arguements
object to count the arguments, but the typeof
operator is not reliable and it'll end up looking ugly and difficult to understand; you'd have to write comments to clarify what you're checking. With overloading you solve this issue.
Here's a more portable/maintainable approach for a jQuery-like library:
https://gist.github.com/elclanrs/5341331
Nice snippet. Here's a shorter version:
String.prototype.template = function() {
var args = arguments;
return this.replace(/\{([^}]+?)\}/g, function(_, match) {
return args[match];
});
};
It's probably because console.log
is not supported on wp7. You can use a polyfill http://paulirish.com/2009/log-a-lightweight-wrapper-for-consolelog/. Why would you log anything in production anyway?
Achievements
331 Karma
12,560 Total ProTip Views
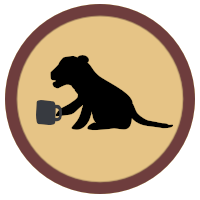
Kona
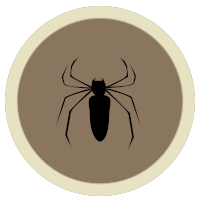
Nephila Komaci
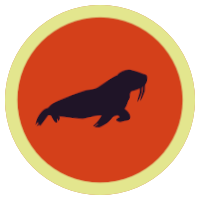
Walrus
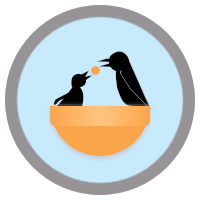
Charity
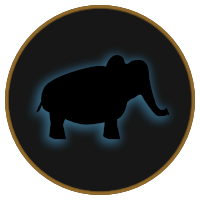
Forked 100
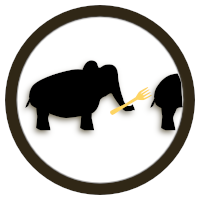
Forked 50
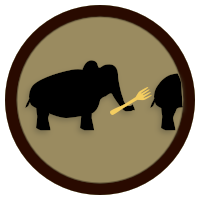
Forked 20
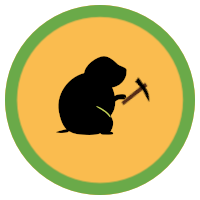
Lemmings 100
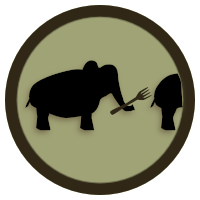
Forked
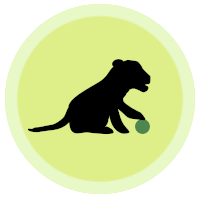
What if there is more than one element with same class? You'd have to use
querySelectorAll
, and loop, just likegetElementsByClassName
. For id's I don't think it's even worth usingquerySelector
, no real gains there, id's are unique. A better example wherequerySelectorAll
is really useful, querying selectors: