Joined May 2011
·
Posted to
Why never use new Array in Javascript
over 1 year
ago
@jjperezaguinaga are you sure that the Array constructor doesn't return Array instances?
[1,2,3] === [1,2,3] // --> false
Array.isArray([1,2,3]) // --> true
Array.isArray(new Array(1,2,3)) // --> true
Also, while I never use the constructor format to initialize my array values, it's very useful for creating medium sized arrays quickly. Suppose I need to create a 10 length array quickly. It's much faster in most engines to do:
var arr = new Array(100);
for (var i = 0; i < 100; i++) {
arr[i] = i * i;
}
vs
var arr = [];
for (var i = 0; i < 100; i++) {
arr.push(i * i);
}
..b.ecause while arrays can resize on the fly, that isn't without cost. It does have to reallocate memory when the size required exceeds the backing store.
Achievements
266 Karma
3,309 Total ProTip Views
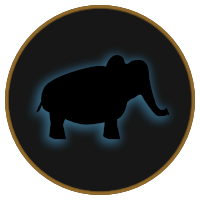
Forked 100
Have a seriously badass project that's been forked at least 100 times
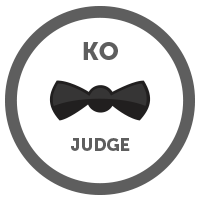
KO Judge
Official Judge of the 2012 Node Knockout
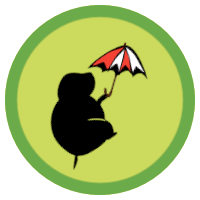
Kilo of Lemmings
Establish a space in the open source hall of fame by getting at least 1000 devs to watch a project
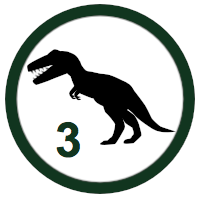
T-Rex 3
Have at least three original repos where C is the dominant language

["KO Innovation", "ko-most-innovative-2011.png", "Won the most innovative app in the 2011 Node Knockout", "winning the most innovative app in the 2011 Node Knockout"]
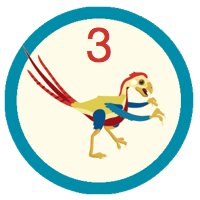
Epidexipteryx 3
Have at least three original repo where C++ is the dominant language
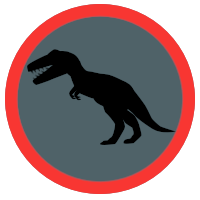
T-Rex
Have at least one original repo where C is the dominant language
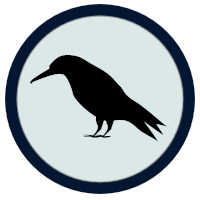
Raven
Have at least one original repo where some form of shell script is the dominant language
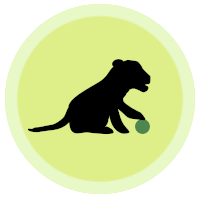
Cub
Have at least one original jQuery or Prototype open source repo
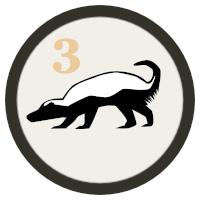
Honey Badger 3
Have at least three Node.js specific repos
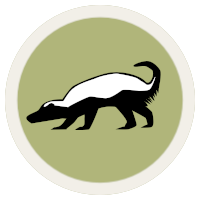
Honey Badger
Have at least one original Node.js-specific repo
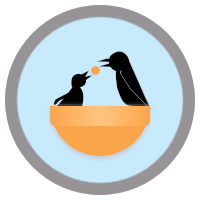
Charity
Fork and commit to someone's open source project in need
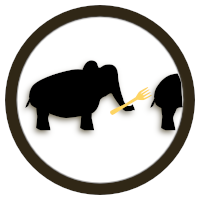
Forked 50
Have a project with a thriving community of users that's been forked at least 50 times
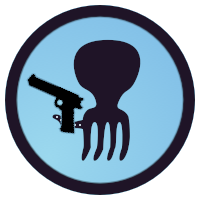
Octopussy
Have a repo followed by a member of the GitHub team
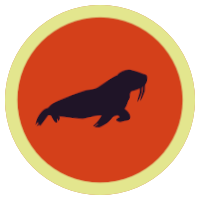
Walrus
The walrus is no stranger to variety. Use at least 4 different languages throughout all your repos
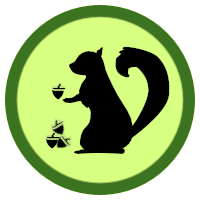
Philanthropist
Truly improve developer quality of life by sharing at least 50 individual open source projects
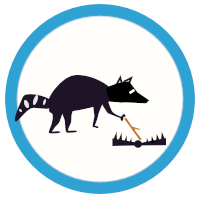
Altruist
Increase developer well-being by sharing at least 20 open source projects
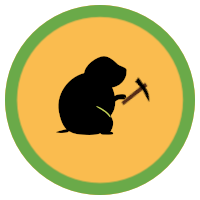
Lemmings 100
Write something great enough to have at least 100 watchers of the project
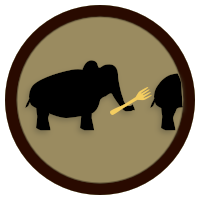
Forked 20
Have an established project that's been forked at least 20 times
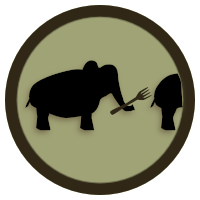
Forked
Have a project valued enough to be forked by someone else
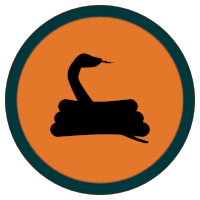
Python
Would you expect anything less? Have at least one original repo where Python is the dominant language
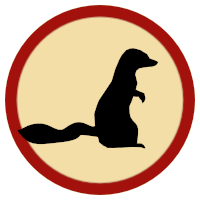
Mongoose
Have at least one original repo where Ruby is the dominant language
@jjperezaguinaga, I promise you that
Array
instances are normal objects just like any other object in javascript. Semantically they work just likeObject
instances except they have a magic.length
property and the addition of a bunch of array methods like.forEach()
,.map()
,.join()
, etc.Arrays are stored by reference just like any other non primitive value. They are mutable. If two variables point to the same array then when the array is changed through one reference, the other reference will see the change since it is the same object.
Regarding using the constructor form with numerical lengths, I find this very useful. There are many cases that I need to quickly create an array of a known size and fill it with data that I get through a loop. For example, to implement
Array.prototype.map
in ES3 browsers, I would do:It would be silly and slow to create an empty array and grow it using
.push()
. While it's technically true that setting values outside the array length also cause it to grow, the VMs are able to often optimize the backing store to the array and speed things up when you declare up front how large it is to be.