Joined June 2012
·
Posted to
Easy JSON (un)marshalling in Scala with Jackson
over 1 year
ago
Is there a way to do this without the: ... .experimental.ScalaObjectMapper
?
Tried using ObjectMapper
, based on this post, but ran into complications with: mapper.readValue[T](json)
:(
Achievements
40 Karma
0 Total ProTip Views
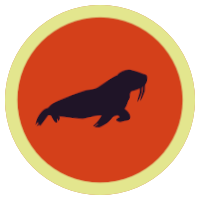
Walrus
The walrus is no stranger to variety. Use at least 4 different languages throughout all your repos
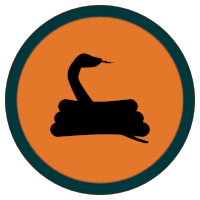
Python
Would you expect anything less? Have at least one original repo where Python is the dominant language
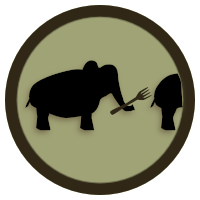
Forked
Have a project valued enough to be forked by someone else
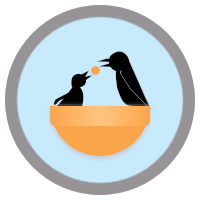
Charity
Fork and commit to someone's open source project in need
Had to simplify, but got it to work this way:
And then can parse to a map using:
JsonUtil.fromJson[Map[String, List[Map[String, String]]]](jsonStr1)
for:
//{"key": "value"}
JsonUtil.fromJson[Map[String, List[Map[String, String]]]](jsonStr2)
for:
//{"key": [ "subKey": "val" ... ]}