Joined March 2012
·
Posted to
Transforming JSON
over 1 year
ago
One might wonder of what use this is. I mean, we've gotten by so far without providing any callbacks, right? One use case that I find myself using the optional callbacks are when a cycle is defined in the JSON object. Consider the following JSON:
let o = {
k1: 'key1',
k2: o
};
You'll notice that k2
points back to the original object, o
. Some platforms will automatically detect this and eliminate the cyclic key (k2
in this case).
Alternatively, you could provide a callback to stringify
to detect said cycle and remove the key yourself:
let seen = [];
JSON.stringify(o, (key, val) => {
if (val && typeof(val) === 'object') {
if (seen.includes(val)) {
return; // Uh-oh, a cycle
}
// Record this value to ensure we avoid serializing it again
seen.push(val);
}
return val;
});
Note: I didn't actually run the code above, so errors may exist.
Achievements
70 Karma
0 Total ProTip Views
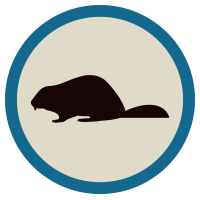
Beaver
Have at least one original repo where go is the dominant language
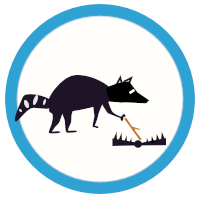
Altruist
Increase developer well-being by sharing at least 20 open source projects
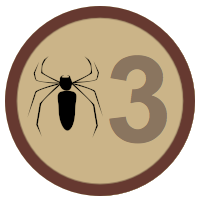
Nephila Komaci 3
Have at least three original repos where PHP is the dominant language
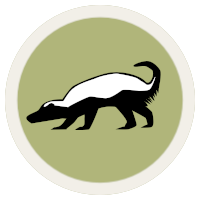
Honey Badger
Have at least one original Node.js-specific repo
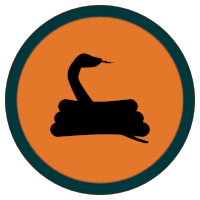
Python
Would you expect anything less? Have at least one original repo where Python is the dominant language
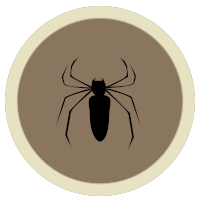
Nephila Komaci
Have at least one original repos where PHP is the dominant language
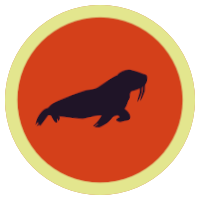
Walrus
The walrus is no stranger to variety. Use at least 4 different languages throughout all your repos
If you're interested in a signed alternative, consider a simple example using bitwise operators: