Interestingly, this doesn't work for me. OPTION+LEFT
still gives [D
, OPTION+RIGHT
still gives [C
. Are there any other possible sources of conflict beyond the general keyboard settings and profile keys?
@jaydeesigns sorryfor leaving this reply late — writing this here for posterity in case anyone else faces the same problem.
Yup, one of the tradeoffs is you have to obscure whatever useful parts of the input are there too. It's worth noting that feedback on the selected file is not at all standardized, but you can extract whatever the browser will allow using the following Javascript (where fileInput
is a reference to the file input):
fileInput.value;
If I'd selected the file at D:\some\thing\foo.bar
the output would be:
Firefox: 'foo.bar',
Chrome: 'C:\fakepath\foo.bar',
IE: 'D:\some\thing\foo.bar'
If you wanted to standardise that, you can extract the only reliable bit (the file name) by applying a regular expression which strips out any sequence of characters followed by a slash:
fileInput.value.replace(/([^\\]*\\)*/,'');
In such a way, you could update the label text (for example), by using jQuery:
$( '.fileContainer [type=file]' ).on( 'click', function updateFileName( event ){
var $input = $( this );
setTimeout( function delayResolution(){
$input.parent().text( $input.val().replace(/([^\\]*\\)*/,'') )
}, 0 )
} );
Note I didn't use the change
event above because IE will only fire that once the user has navigated (tabbed or clicked) away from the input after using it. Instead we listen for the click
event (which will trigger on keyboard activation too), and use a setTimeout
to make the function resolve as soon as possible (zero milliseconds): in this way, the user clicks, the dialogue has time to open, and Javascript only executes when the user has finished inputting.
Achievements
947 Karma
295,018 Total ProTip Views
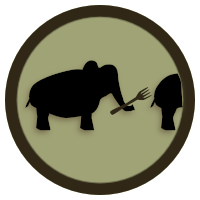
Forked
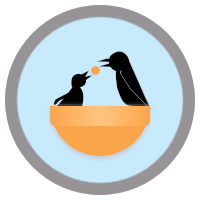
Charity
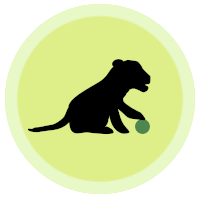
The void expression isn't necessary: the anonymous function scope instantiates a new
undefined
argument, and not supplying anything is terser than usingvoid
:But if, like me, you're really not keen on closing parentheses lines away from their opening counterpart,
void
is useful as a way of executing a closure (without that pair of parentheses!):BTW
void
is an operator, not a function, so you don't need the parens — you can just writevoid 0
:)