I was under the impression, somehow, that whilst the selector engine does work right to left that it does optimise easy stuff like ID selection?
That said, for less generic cases, minimising the search space is definitely a good idea. You can also compact it a little by doing jQuery('a', '#foo')
Improved version that still has the weighting towards the start:
function generate_string($length = 6, $variance = 3, $weight = 2) {
$length += rand(0 - $variance, $variance);
$l0 = str_split('tashwiobmfcldpnegryuvjkqxz');
$l = str_split('etaoinshrdlcumwfgypbvkjxqz.');
$str = '';
for ($i = 0; $i < $length; $i++) {
$a = $i == 0 ? $l0 : $l;
$len = count($a) - 1;
$s = ceil($len - ($len * log(rand(0, $len), $len)));
$str .= $a[$s];
}
return $str;
}
Timings:
Dark: 0.12173390388489
Rich:0.15515804290771
Something like the following provides a good weighted value, which can be modified by changing 2 to something larger/smaller (within reason).
$char = $len - round(log(pow(rand(0,$len),2))*$len*(1/2))
That would almost definitely be faster, even with the log.
Mine has the inner loop for weighting towards the start of the range - this could be done more efficiently (and more tunable) with logs or similar, but really any weighting will introduce a cost.
Ultimately, for test data, "human-ness" is pretty unimportant. And if you want to get it looking even close to reasonable, you need to use markov chains.
I made something similar a few days ago, with the added feature of generating strings with a high tendency to include more common characters.
function generate_string($length = 7, $variance = 5, $weight = 3) {
$length += rand(0 - $variance, $variance);
$l0 = str_split('tashwiobmfcldpnegryuvjkqxz');
$l = str_split('etaoinshrdlcumwfgypbvkjxqz.');
$str = '';
for ($i = 0; $i < $length; $i++) {
$a = $i == 0 ? $l0 : $l;
$s = count($a);
for ($r = 1; $r < $weight; $r++) {
$s = min(count($a) - 1, rand(0, $s));
}
$str .= $a[$s];
}
return $str;
}
It's used for generating test data so the code is fairly shoddy (especially variable naming), but the output is solid.
@couto we are using this function in a Node.js environment, so browser support isn't a consideration; having a debug/log function simply being rewritten to an empty one is the better choice in this case because when something breaks we can just turn the debugging output back on to track it down.
In a browser environment, as you said, console.log breaks IE. By wrapping in a debug/log function you can guard against exceptions by checking for the existence of console.log.
ah, that does sound quite useful; we're working in Node.js for ours so directly using later isn't possible, but it can definitely form the basis for the next rewrite of the queue system!
@couto not "just" that I have a nice function: with proper debugging/logging tools (ie, not relying on raw console.log) you can easily do stuff like filtering what logs you see, or replace the debug/log function with an empty fn for production mode. Thus making a tool like groundskeeper fairly redundant.
@erol what is the benefit of avoiding setnx? I assume it does a key lookup before write which is two ops instead of one.
the zset queue seems to be a more natural solution, perhaps you could do a writeup?
@mdeiters yep, Beanstalkd is now completely out of our dependencies list!
We have a global "debug" function that wraps up a bunch of nice features, like safely printing circular objects, "highlighting" certain debug messages, and pretty-printing everything.
On top of that, we have a "show stack" method to print out the stack trace up to that point.
Here it is as a gist: https://gist.github.com/3796687
Achievements
498 Karma
36,430 Total ProTip Views
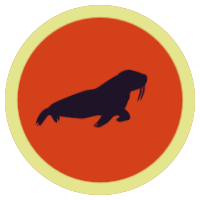
Walrus
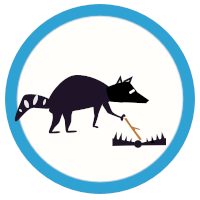
Altruist

Nephila Komaci
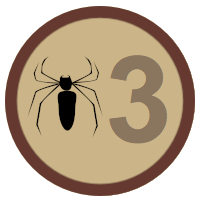
Nephila Komaci 3

Forked
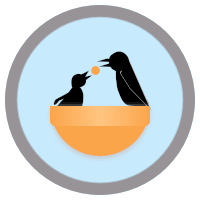
Charity
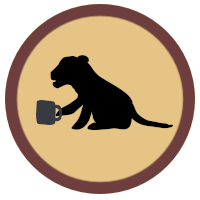
If the asset_path is an issue, you can define a custom function for the images to work around it (although this removes the ability to configure with config.rb):
@function remote-image-url($name) {
@return unquote("url(http://your.domain.com" + image-url($path) + ")");
}
Then just call remote-image-url instead of image-url when you want the path in there!